Please read my previous article :
Visual Studio provides some auto-generated code. Let's delete the IService1.cs and Service1.svc files. Add a WCF service file and name it MyService.svc and provide it the following code. It adds the following two files.
- IMyService.cs
- MyService.svc
- using System.ServiceModel;
- namespace myFirstApp
- {
- [ServiceContract]
- public interface IMyService
- {
- [OperationContract]
- int AddTwoNo(int intFirstNo, int intSecondNo);
- }
- }
OperationContract is defined within a ServiceContract. It defines the parameters and return type of the operation.
Now implement the ImyService interface in MyService.svc.cs. Let's delete all the code first from MyService.svc.cs and write the following:
- namespace myFirstApp
- {
- public class MyService : IMyService
- {
- public int AddTwoNo(int intFirstNo, int intSecondNo)
- {
- return intFirstNo + intSecondNo;
- }
- }
- }
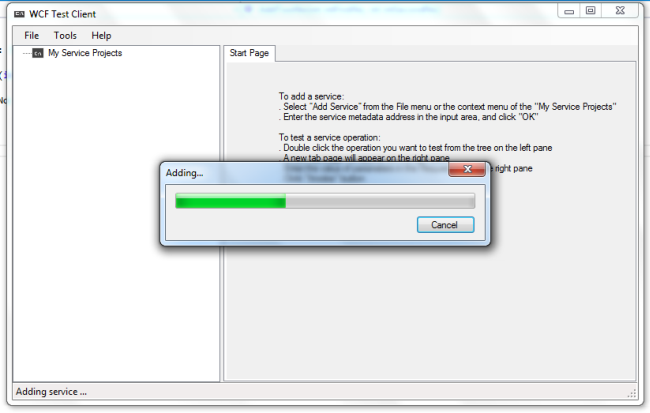
After running it successfully double-click on AddTwoNo() and fill in the value in intFirstNo and intSecondNo respectively. I am putting 5 and 6 and hitting the invoke button.
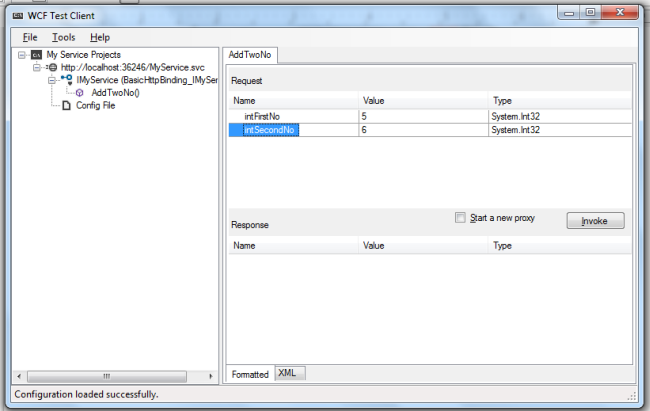
Output:
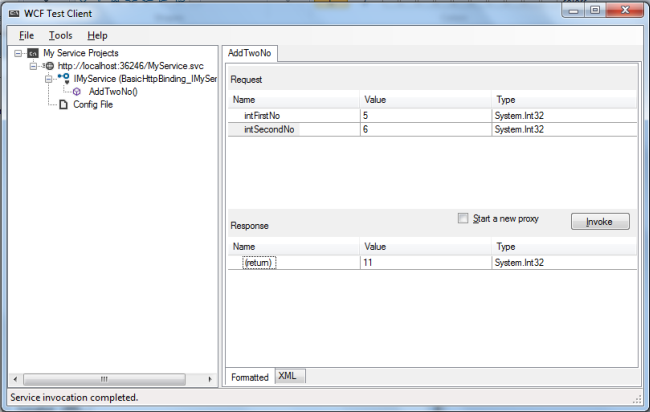
Note: The default binding in WCF is basicHttpBinding. We can see all details of this service at http://localhost:36246/myservice.svc that is shown in the preceding image.
Now I am calling this WCF service using an ASP.NET application.
Open a new Visual Studio instance and go to "File" -> "New" -> "Project..." and select ASP.NET Empty Web Application and name it WCFClientApp.
Add a webform and name it default.aspx and add the service reference.
Right-click on the project (WCFClientApp).
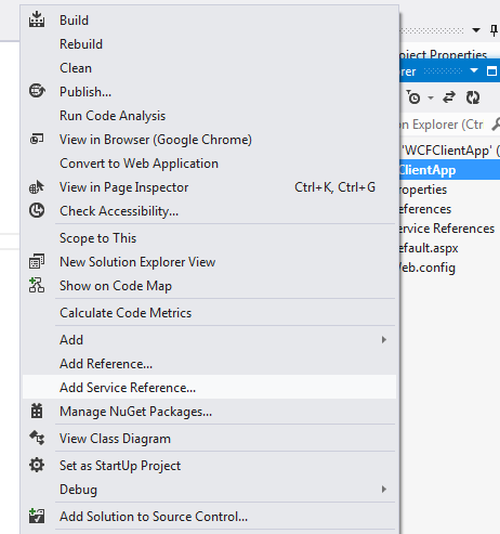
Click on Add service reference and fill in the address and click go. Then change the namespace as you want and click OK. I am keeping the WCFReference namespace.
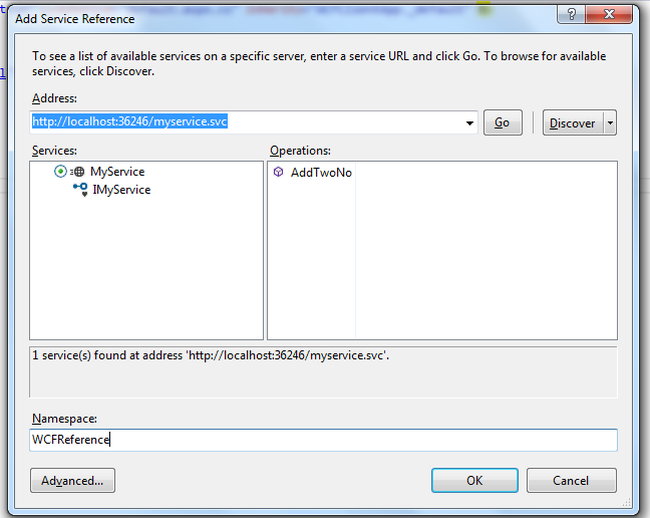
In default.aspx
- <table>
- <tr><td>First No</td><td><asp:TextBox ID="txtFirst" runat="server"></asp:TextBox></td></tr>
- <tr><td>Second No</td><td><asp:TextBox ID="txtSec" runat="server"></asp:TextBox></td></tr>
- <tr><td colspan="2"><asp:Button ID="btnAdd" runat="server" Text="Add" OnClick="btnAdd_Click" /></td></tr>
- <tr><td colspan="2"><asp:Label ID="lblResult" runat="server"></asp:Label></td></tr>
- </table>
- using System;
- namespace WCFClientApp
- {
- public partial class _default : System.Web.UI.Page
- {
- protected void btnAdd_Click(object sender, EventArgs e)
- {
- int intFirstNo = 0, intSecNo = 0, intResult = 0;
- intFirstNo = Convert.ToInt16(txtFirst.Text);
- intSecNo = Convert.ToInt16(txtSec.Text);
- WCFReference.MyServiceClient client = new WCFReference.MyServiceClient();
- intResult = client.AddTwoNo(intFirstNo, intSecNo);
- lblResult.Text = "Result is :"+intResult;
- }
- }
- }
Output
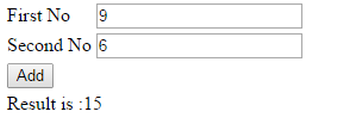
I hope this article is helpful for you.
If you are searching life partner. your searching end with kpmarriage.com. now kpmarriage.com offer free matrimonial website which offer free message, free chat, free view contact information. so register here : kpmarriage.com- Free matrimonial website
0 comments:
Post a Comment