This code example demonstrates how to export data from DataGridView control to Excel document using C#.
Step 1: Add a reference to the ‘Microsoft.Office.Interop’ to your project from .NET components.
Step 2: Creating Excel Application
Step 3: Change properties of the Workbook
Step 4: Storing header part in Excel
Step 5: Storing Each row and column value to excel sheet
Step 6: Save Workbook and exit
Windows Forms Layout
and excel file after exporting
Complete Source Code
Step 1: Add a reference to the ‘Microsoft.Office.Interop’ to your project from .NET components.
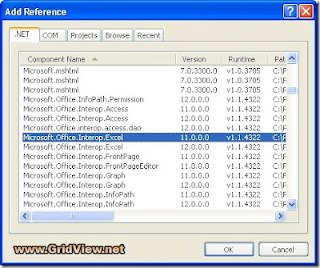
Step 2: Creating Excel Application
Microsoft.Office.Interop.Excel.ApplicationClass ExcelApp = new Microsoft.Office.Interop.Excel.ApplicationClass();
ExcelApp.Application.Workbooks.Add(Type.Missing);
Step 3: Change properties of the Workbook
ExcelApp.Columns.ColumnWidth = 20;
Step 4: Storing header part in Excel
for (int i = 1; i < dataGridView1.Columns.Count + 1; i++)
{
ExcelApp.Cells[1, i] = dataGridView1.Columns[i - 1].HeaderText;
}
Step 5: Storing Each row and column value to excel sheet
for (int i = 0; i < dataGridView1.Rows.Count - 1; i++)
{
for (int j = 0; j < dataGridView1.Columns.Count; j++)
{
ExcelApp.Cells[i + 2, j + 1] = dataGridView1.Rows[i].Cells[j].Value.ToString();
}
}
Step 6: Save Workbook and exit
ExcelApp.ActiveWorkbook.SaveCopyAs("C:\\test.xls");
ExcelApp.ActiveWorkbook.Saved = true;
ExcelApp.Quit();
Windows Forms Layout
and excel file after exporting
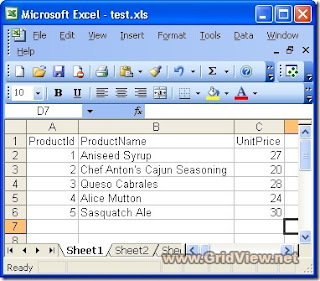
Complete Source Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using Microsoft.Office.Interop;
namespace Export_DataGridView_to_Excel
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
DataSet ds = new DataSet();
ds.ReadXml("C:\\Products.xml");
dataGridView1.DataSource = ds.Tables[0];
}
private void button1_Click(object sender, EventArgs e)
{
Microsoft.Office.Interop.Excel.ApplicationClass ExcelApp = new Microsoft.Office.Interop.Excel.ApplicationClass();
ExcelApp.Application.Workbooks.Add(Type.Missing);
// Change properties of the Workbook
ExcelApp.Columns.ColumnWidth = 20;
// Storing header part in Excel
for (int i = 1; i < dataGridView1.Columns.Count + 1; i++)
{
ExcelApp.Cells[1, i] = dataGridView1.Columns[i - 1].HeaderText;
}
// Storing Each row and column value to excel sheet
for (int i = 0; i < dataGridView1.Rows.Count - 1; i++)
{
for (int j = 0; j < dataGridView1.Columns.Count; j++)
{
ExcelApp.Cells[i + 2, j + 1] = dataGridView1.Rows[i].Cells[j].Value.ToString();
}
}
ExcelApp.ActiveWorkbook.SaveCopyAs("C:\\test.xls");
ExcelApp.ActiveWorkbook.Saved = true;
ExcelApp.Quit();
}
}
}
If you are searching life partner. your searching end with kpmarriage.com. now kpmarriage.com offer free matrimonial website which offer free message, free chat, free view contact information. so register here : kpmarriage.com- Free matrimonial website
0 comments:
Post a Comment